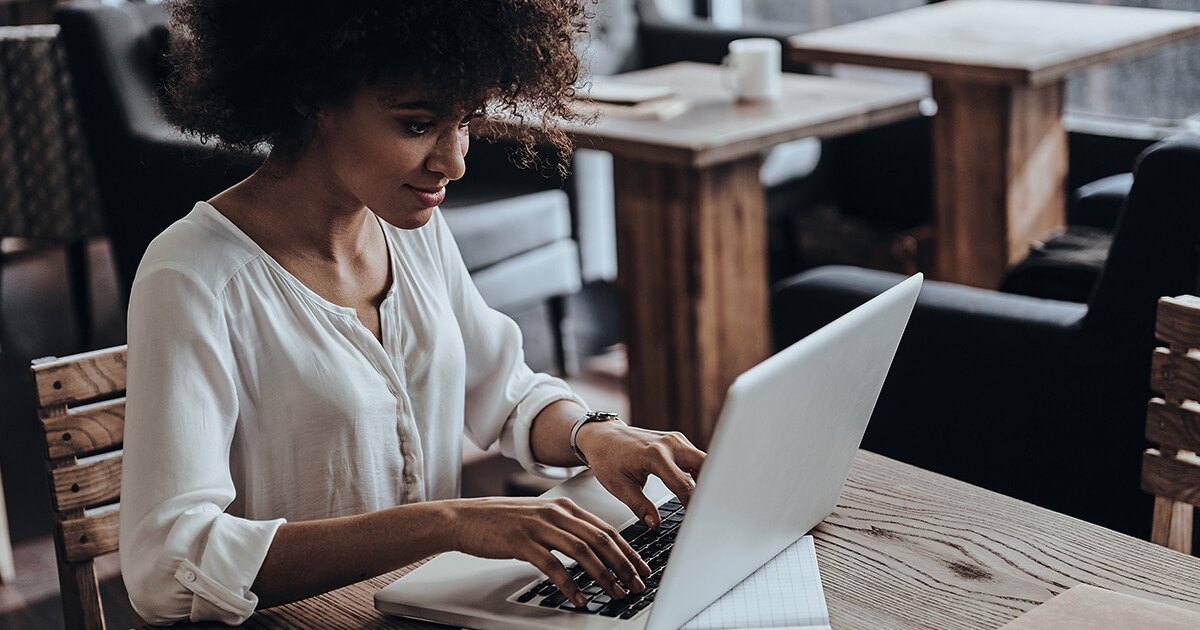
In the Python Internet programming language, the dictionary (or dictionary) is an important data type that allows you to relate terms to meanings. By using key-value pairs, you can create as many tables, data collections or inventories. A Python Dictionary can also be used with Python for loops, Python if-else statements, and Python while loops.
Free IONOS API
Use the IONOS API at no additional cost to retrieve or update your domain, DNS and SSL data.



What is a Python Dictionary?
A Python Dictionary is a data type that associates terms and meanings. In this structure, the keys (keys) and values (values) can be associated, saved and later retrieved. If we sometimes find the awkward expression of « associative list » in French to designate a Python Dictionary, we will prefer the more eloquent translation of « dictionary ». As in a dictionary, in which a term is associated with an explanation or a translation, a mapping table is also created in a Python Dictionary and cannot contain duplicates. Each key appears only once.
Python Dictionary: structure and query
A Python Dictionary is always surrounded by braces. The key and its associated value are connected by a colon and the resulting pairs are separated by commas. In theory, a Python Dictionary can contain an infinite number of key-value pairs. The key is in turn enclosed in quotes. You’ve probably already learned about the syntax in a Python tutorial. Its wording may look like this:
ages = {'Jim': 42, 'Jack': 13, 'John': 69}
The Python Dictionary is read by enclosing the correct key in square brackets. The code is written as follows:
age_jim = ages['Jim']
assert age_jim == 42
Python Dictionary Example
A simple example illustrates the structure and operation of the Python Dictionary. The following code is to affect and exit countries and their capitals. The Python Dictionary looks like this:
capitals = { "UK": "London", "FR": "Paris", "DE": "Berlin" }
To query for a specific element now, use the following hooks:
capitals["FR"]
assert capitals["FR"] == 'Paris'
In this case, the output corresponds to the value belonging to the key “France”, that is to say “Paris”.
Alternate structure
You can also create a Python Dictionary with no content and then populate it afterwards. To do this, create an empty Python Dictionary that looks like this:
Now fill in the dictionary:
capitals["UK"] = "London"
capitals["FR"] = "Paris"
capitals["DE"] = "Berlin"
If you now query the « capital » Python Dictionary, you will get the following output:
{ "UK": "London", "FR": "Paris", "DE": "Berlin" }
Edit the Python Dictionary later
You can also modify your Python Dictionary afterwards. For example, you can write this to append key-value pairs:
capitals[ "IT" ] = "Roma"
print(capitals)
The corresponding output is now:
{ "UK": "London", "FR": "Paris", "DE": "Berlin", "IT": "Roma" }
If you want to modify a value in a key-value pair, use the following code:
capitals = { "UK": "London", "FR": "Paris", "DE": "Berlin", "IT": "???" }
assert capitals["IT"] == "???"
capitals["IT"] = "Roma"
assert capitals["IT"] == "Roma"
The output will then again be as follows:
{ "UK": "London", "FR": "Paris", "DE": "Berlin", "IT": "Roma" }
Remove key-value pairs from Python Dictionary
There are three ways to remove a key-value pair from the Python Dictionary: del, pop, and popitem.
delete with del
A simple inventory can be used to illustrate the del method. Suppose a bakery offers 100 rolls, 25 loaves and 20 croissants when it opens. The corresponding Python Dictionary would then look like this:
stock = { "rolls": 100, "breads": 25, "croissants": 20 }
If the bakery is now selling all the croissants and wants to remove them from its list, it calls the del function for this purpose:
del stock["croissants"]
print(stock)
However, this method imposes the systematic use of a key-value pair in relation to the del command. Otherwise, the entire Python Dictionary would be deleted. This Python problem appears relatively frequently.
delete with pop
The second way to remove key-value pairs from a Python Dictionary is the pop method. This allows you to save the deleted value to a new variable. This works as follows:
marbles = { "red": 13, "green": 19, "blue": 7, "yellow": 21}
red_marbles = marbles.pop("red")
print(f"We've removed {red_marbles} red marbles.")
print(f"Remaining marbles are: {marbles}")
The output then looks like this:
We've removed 13 red marbles.
Remaining marbles are: {'green': 19, 'blue': 7, 'yellow': 21}
Delete with popitem
Use popitem to remove the last key-value pair from your Python Dictionary. Here is the relevant code:
last = marbles.popitem()
print(last)
print(marbles)
Your output then looks like this:
(‘yellow’, 21)
{ « One »: 1, « Two »: 2, « Three »: 3 }
Other methods for the Python Dictionary
Other methods that work with a Python Dictionary are available to you. Here is a list of the main options:
Method |
Description |
---|---|
clear |
clear removes all key-value pairs from the Python Dictionary. |
copy |
copy creates a copy of the dictionary in memory. |
get |
get allows the value to be determined by entering the key. |
keys |
keys allows you to read your dictionary. |
update |
update allows you to supplement one Python Dictionary with another. Redundant keys are overwritten in the added dictionary. |
values |
values allows you to query all values in your dictionary. |