With the Java BigDecimal class, it is possible to process complex floating point numbers precisely. These must first be created, and can then be used by various methods. In doing so, the syntax used is always structured in a similar way, so that beginners can use this class after a short learning period.
What is Java BigDecimal?
Java BigDecimal is a class that allows you to represent and manipulate complex floating point numbers of any size in theory with high precision. Using various methods that we present to you in this article, you can perform rounding, arithmetic, format conversion, hashing, and advanced comparison operations of the highest precision with this class.
Java BigDecimal consists of a 32-bit integer scale and a unscaled integer value (unscale in English) of any precision. In this case, « scale » refers to the number of digits after the decimal point, if it is greater than or equal to zero. If the value is less than zero, however, it is multiplied by « 10^(-scale) ». The possible size of the class is limited only by the computer's memory. This aspect remains rather theoretical because it is unlikely that a program will create a number that exceeds the available memory. While BigDecimal in Java is intended exclusively for floating point numbers, integers are handled with the BigInteger class.
Web hosting
Flexible, high-performance and secure web hosting
- SSL Certificate and DDoS Protection
- Data Backup and Restore
- 24/7 assistance and personal advisor
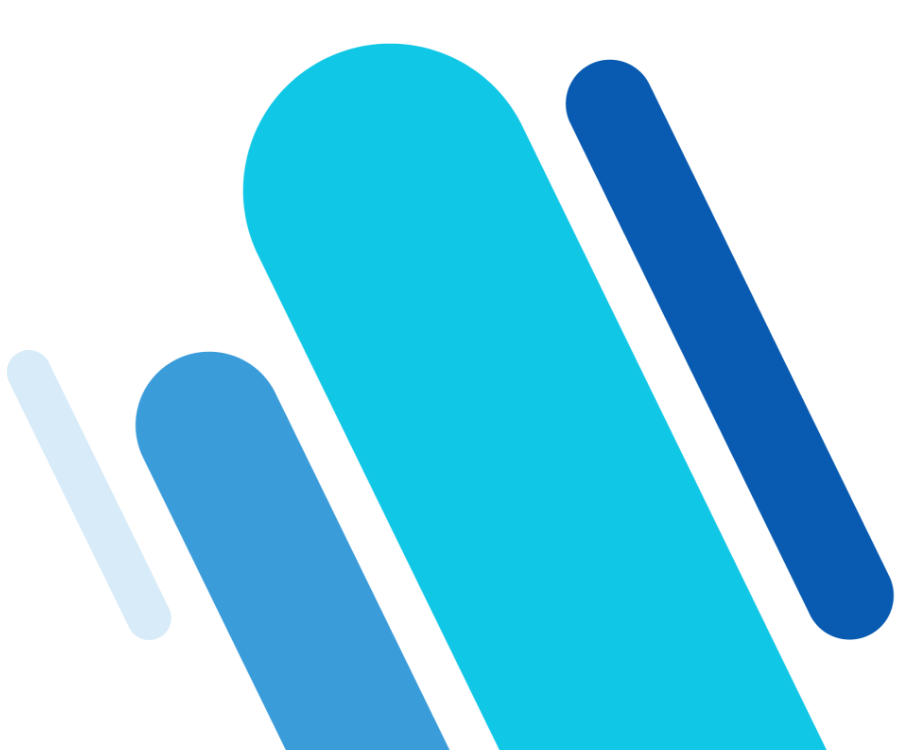
What is the class for?
Due to its precision, Java BigDecimal is not required for every application, but there are situations and application areas where the class is indispensable. Calculations where even the smallest decimal can have a significant effect occur, for example, in the field of e-commerce for transactions. The class is also used for precise static analyses. Many programs, which are used for example for the the control and navigation of aircraft or missilesrely on this class. Java BigDecimal is also used in the medical domain. In other sectors, this precise calculation offers the greatest possible certainty.
If you want to use Java BigDecimal, you must first import the class into the Java program. Once this is done, you can then declare an object of this classthen create the desired value as an argument and pass it to the appropriate Java constructor. Once this is done, you can use the BigDecimals created in Java. There are several methods available within the class, the usage of which we detail in the following sections. Here's how to import the class first and then declare two example objects:
/ / Votre programme Java pour la classe BigDecimal
import java.math.BigDecimal;
public class BigDecimalExemple
{
public static void main(String[] args)
{
/ / Créer deux nouveaux BigDecimal
BigDecimal ExempleUn =
new BigDecimal ("1275936001.744297361");
BigDecimal ExempleDeux =
new BigDecimal ("4746691047.132719503");
}
}
Java
You can now use these objects with the corresponding methods of the class.
Examples for Java BigDecimal
After creating the objects, you use different methods to perform operations and use the objects in this way. We will show you with some simple examples how this works and what results you can get. The output is initiated by the Java command System.out.println()
.
Add two BigDecimals
If you want to add two BigDecimals in Java, you can use the method add(). To do this, you fill in the two values whose sum you want to calculate. In our example, we want to add the value « ExampleOne » to the value « ExampleTwo ». The code looks like this:
ExempleUn =
ExempleUn.add(ExempleDeux);
System.out.println ("Le résultat recherché après l’addition est : " + ExempleUn);
Java
Subtract numbers
To subtract two values you need the method subtract(). The following example involves subtracting « ExampleTwo » from « ExampleOne ». The corresponding code looks like this:
ExempleUn =
ExempleUn.subtract(ExempleDeux);
System.out.println ("Le résultat recherché après la soustraction est : " + ExempleUn);
Java
Multiply the values
The method by which you multiply two BigDecimals in Java works very similarly. It is multiply() and uses the « multiply » object as an argument. If you want to multiply « ExampleTwo » by « ExampleOne », the corresponding code looks like this:
ExempleUn =
ExempleUn.multiply(ExempleDeux);
System.out.println ("Le résultat recherché après la multiplication est : " + ExempleUn);
Java
Dividing numbers
If you want to divide two BigDecimals in Java, go for the method divide(). In principle, it also adopts the known syntax and looks like this:
ExempleUn =
ExempleUn.divide(ExempleDeux);
System.out.println ("Le résultat recherché après la division est : " + ExempleUn);
Java
This only works, however, if the result is exact or an integer. If this is not the case, the following error message is issued: java.lang.ArithmeticException: Non-terminating decimal expansion; no exact representable decimal result.
. This describes a runtime error. To avoid this, there are different rounding options for the divide() method, which can be passed via java.math.RoundingMode. You can choose from the following constants:
Constant | Function |
---|---|
CEILING | Rounds to positive infinity |
DOWN | Rounds to 0 |
FLOOR | Round to negative infinity |
HALF_DOWN | Rounds to the nearest neighbor and opposite to 0, if the two are equidistant |
HALF_EVEN | Rounds to the nearest neighbor and to the nearest even number, if the two are equidistant |
HALF_UP | Rounds to nearest neighbor and in the 0 direction, if both are equally distant |
UNNECESSARY | Does not require rounding and performs only exact operations. This constant can only be used if the division is exact |
UP | Rounds from 0 |
Overview of the most important methods
Now that you have learned how to use Java BigDecimal and call methods for this purpose, we will conclude by presenting some of the most important methods in an overview.
Method | Function |
---|---|
abs() | BigDecimal with the absolute value of that BigDecimal |
add() | Returns a BigDecimal whose value is composed of (this + Addend) |
divide() | The output value results from (this / Divisor) |
max(BigDecimal val) | Returns the maximum of this BigDecimal |
min(BigDecimal val) | Returns the minimum of this BigDecimal |
movePointLeft(int n) | Returns a BigDecimal where the decimal point has been shifted to the left by the value 'n'. |
movePointRight(int n) | Returns a BigDecimal where the decimal point has been shifted to the right by the value 'n'. |
multiply(BigDecimal multiplicand, MathContext mc) | Returns a value resulting from (this * multiplicand) |
Advice
Our Digital Guide allows you to learn much more about the well-known and popular programming language: for example, you can get acquainted with Java operators and discover the essentials about the differences between Java and JavaScript or Java and Python.